31. Relations#
You can model relationships between content items by placing them in a hierarchy (e.g. a folder speakers containing the (folderish) speakers and within each speaker the talks) or by linking them to each other in Richtext fields. But where would you then store a talk that two speakers give together?
Relations allow developers to model relationships between objects without using links or a hierarchy. The behavior plone.app.relationfield.behavior.IRelatedItems
provides the field Related Items in the tab Categorization. That field simply says a
is somehow related to b
.
By using custom relations you can model your data in a much more meaningful way.
31.1. Creating and configuring relations in a schema#
Relate to one item only.
1from z3c.relationfield.schema import RelationChoice
2
3evil_mastermind = RelationChoice(
4 title="The Evil Mastermind",
5 vocabulary="plone.app.vocabularies.Catalog",
6 required=False,
7)
Relate to multiple items.
1from z3c.relationfield.schema import RelationChoice
2from z3c.relationfield.schema import RelationList
3
4minions = RelationList(
5 title="Minions",
6 default=[],
7 value_type=RelationChoice(
8 title="Minions",
9 vocabulary="plone.app.vocabularies.Catalog"
10 ),
11 required=False,
12)
We can see that the code for the behavior IRelatedItems does exactly the same.
Controlling what to relate to#
The best way to control wich item should be relatable to is to configure the widget with directives.widget()
.
In the following example you can only relate to Documents:
1from plone.app.z3cform.widget import RelatedItemsFieldWidget
2
3relationchoice_field = RelationChoice(
4 title="Relationchoice field",
5 vocabulary="plone.app.vocabularies.Catalog",
6 required=False,
7)
8directives.widget(
9 "relationchoice_field",
10 RelatedItemsFieldWidget,
11 pattern_options={
12 "selectableTypes": ["Document", "Event"],
13 },
14)
Define Favorite Locations#
The RelatedItemsFieldWidget
allows you to set favorites:
1directives.widget(
2 "minions",
3 RelatedItemsFieldWidget,
4 pattern_options={
5 "favorites": [{"title": "Minions", "path": "/Plone/minions"}]
6 },
7)
favorites
can also be a method that takes the current context. Here is a full example as a behavior:
1from plone import api
2from plone.app.vocabularies.catalog import CatalogSource
3from plone.app.z3cform.widget import RelatedItemsFieldWidget
4from plone.autoform import directives
5from plone.autoform.interfaces import IFormFieldProvider
6from plone.supermodel import model
7from z3c.relationfield.schema import RelationChoice
8from z3c.relationfield.schema import RelationList
9from zope.interface import provider
10
11
12def minion_favorites(context):
13 portal = api.portal.get()
14 minions_path = "/".join(portal["minions"].getPhysicalPath())
15 one_eyed_minions_path = "/".join(portal["one-eyed-minions"].getPhysicalPath())
16 return [
17 {
18 "title": "Current Content",
19 "path": "/".join(context.getPhysicalPath())
20 }, {
21 "title": "Minions",
22 "path": minions_path,
23 }, {
24 "title": "One eyed minions",
25 "path": one_eyed_minions_path,
26 }
27 ]
28
29
30@provider(IFormFieldProvider)
31class IHaveMinions(model.Schema):
32
33 minions = RelationList(
34 title="My minions",
35 default=[],
36 value_type=RelationChoice(
37 source=CatalogSource(
38 portal_type=["one_eyed_minion", "minion"],
39 review_state="published",
40 )
41 ),
42 required=False,
43 )
44 directives.widget(
45 "minions",
46 RelatedItemsFieldWidget,
47 pattern_options={
48 "mode": "auto",
49 "favorites": minion_favorites,
50 }
51 )
31.2. RelationFields through the web or in xml#
It is surprisingly easy to create RelationFields through the web
Using the Dexterity schema editor, add a new field and select Relation List or Relation Choice, depending on whether you want to relate to multiple items or not.
When configuring the field you can even select the content type the relation should be limited to.
When you click on Edit XML field model
you will see the fields in the XML schema:
RelationChoice:
1<field name="boss" type="z3c.relationfield.schema.RelationChoice">
2 <description/>
3 <required>False</required>
4 <title>Boss</title>
5</field>
RelationList:
1<field name="underlings" type="z3c.relationfield.schema.RelationList">
2 <description/>
3 <required>False</required>
4 <title>Underlings</title>
5 <value_type type="z3c.relationfield.schema.RelationChoice">
6 <title i18n:translate="">Relation Choice</title>
7 <portal_type>
8 <element>Document</element>
9 <element>News Item</element>
10 </portal_type>
11 </value_type>
12</field>
31.3. Using different widgets for relations#
Often the standard widget for relations is not what you want since it can be hard to navigate to the content you want to relate to.
If you want to use checkboxes, radiobuttons or a selection-dropdown you need to use StaticCatalogVocabulary
instead of CatalogSource
to specify your options.
1from plone.app.vocabularies.catalog import StaticCatalogVocabulary
2from plone.app.z3cform.widget import SelectFieldWidget
3from plone.autoform import directives
4from z3c.relationfield.schema import RelationChoice
5
6relationchoice_field_select = RelationChoice(
7 title="RelationChoice with Select Widget",
8 vocabulary=StaticCatalogVocabulary(
9 {
10 "portal_type": ["Document", "Event"],
11 "review_state": "published",
12 }
13 ),
14 required=False,
15)
16directives.widget(
17 "relationchoice_field_select",
18 SelectFieldWidget,
19)
The field should then look like this:
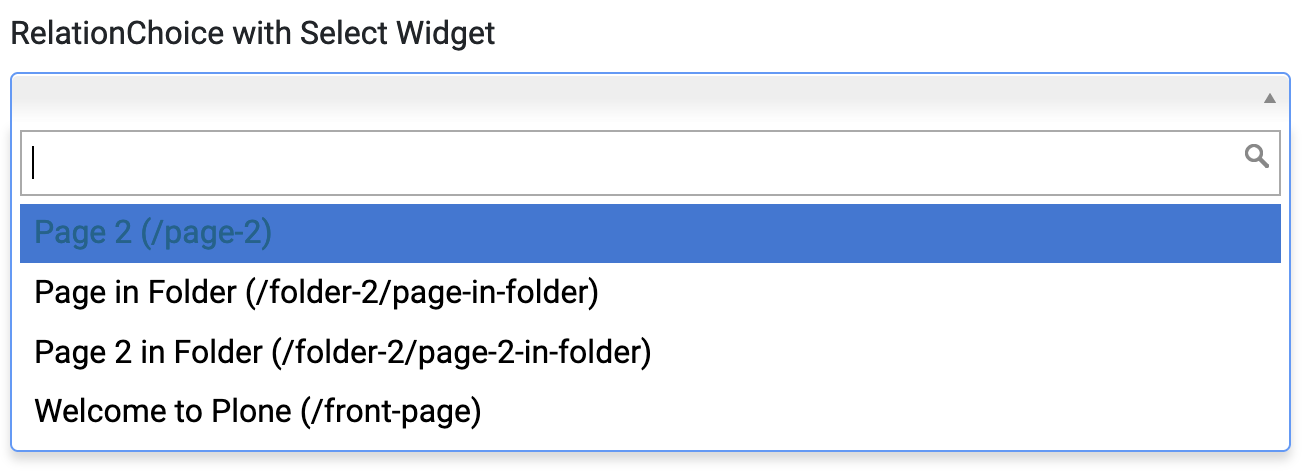
RelationList field with select widget#
Another example is the AjaxSelectFieldWidget
that only queries the catalog for results if you start typing:
1relationlist_field_ajax_select = RelationList(
2 title="Relationlist Field with AJAXSelect",
3 description="z3c.relationfield.schema.RelationList",
4 value_type=RelationChoice(
5 vocabulary=StaticCatalogVocabulary(
6 {
7 "portal_type": ["Document", "Event"],
8 "review_state": "published",
9 }
10 )
11 ),
12 required=False,
13)
14directives.widget(
15 "relationlist_field_ajax_select",
16 AjaxSelectFieldWidget,
17 vocabulary=StaticCatalogVocabulary(
18 {
19 "portal_type": ["Document", "Event", "Folder"],
20 },
21 title_template="{brain.Type}: {brain.Title} at {path}",
22 ), # Custom item rendering
23 pattern_options={ # Options for Select2
24 "minimumInputLength": 2, # - Don't query until at least two characters have been typed
25 "ajax": {"quietMillis": 500}, # - Wait 500ms after typing to make query
26 },
27)
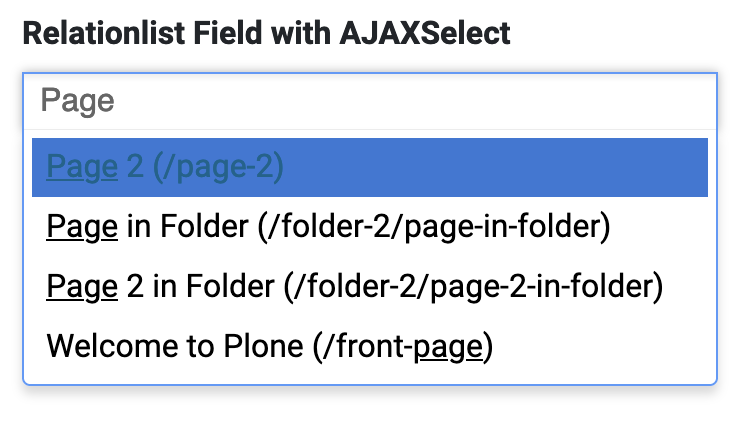
Relationlist Field with AJAXSelect
31.5. Inspecting relations#
You Plone 6 Classic you can inspect all relations and backrelations in your site using the control panel /@@inspect-relations
.
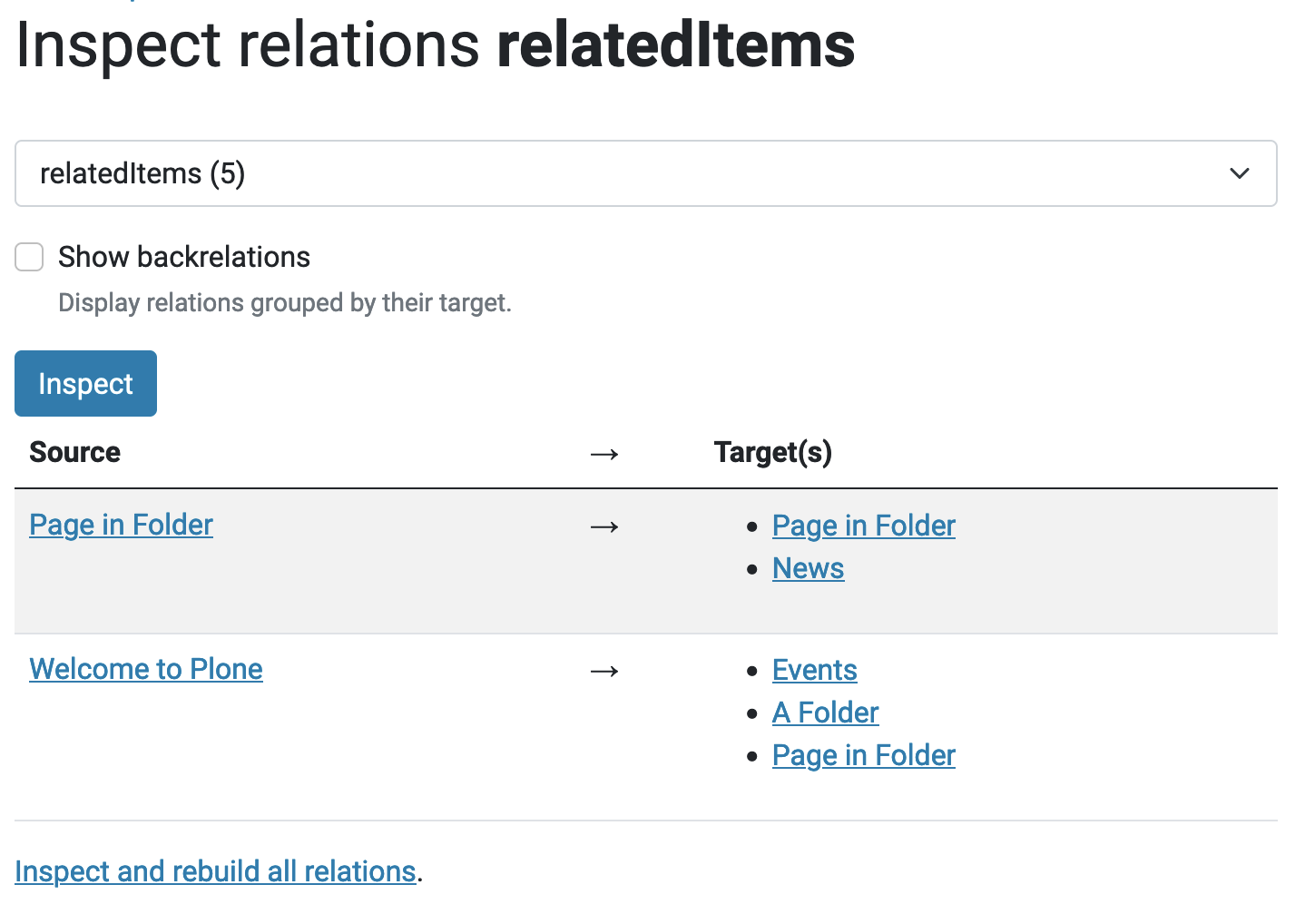
The relations controlpanel#
In Plone 5 this is available through the addon collective.relationhelpers.
31.6. Programming with relations#
Plone 6#
Since Plone 6 plone.api
has methods to create, read, and delete relations and backrelations.
1from plone import api
2
3portal = api.portal.get()
4source = portal["bob"]
5target = portal["bobby"]
6api.relation.create(source=source, target=target, relationship="friend")
1from plone import api
2
3api.relation.get(source=portal["bob"])
4api.relation.get(relationship="friend")
5api.relation.get(target=portal["bobby"])
1from plone import api
2
3api.relation.delete(source=portal["bob"])
See the chapter Relations of the docs for plone.api
for more details.
Plone 5.2 and older#
In older Plone-Versions you can use collective.relationhelpers to create and read relations and backrelations in a very similar way.
Restapi#
A restapi endpoint to create, read, and delete relations and backrelations will be part of plone.restapi
. See plone/plone.restapi#1432
31.7. Relationfields without relations#
A light-weight alternative to using relations is to store a UUID of the object you want to link to. Obviously you will loose the option to query the relation-catalog for these but you could create a custom index for that purpose.
The trick is to use Choice
and List
instead of RelationChoice
or RelationList
and configure the field to use RelatedItemsFieldWidget
:
1from plone.app.z3cform.widget import RelatedItemsFieldWidget
2from plone.autoform import directives
3from zope import schema
4
5uuid_choice_field = schema.Choice(
6 title="Choice field with RelatedItems widget storing uuids",
7 description="schema.Choice",
8 vocabulary="plone.app.vocabularies.Catalog",
9 required=False,
10)
11directives.widget("uuid_choice_field", RelatedItemsFieldWidget)
Again you can use StaticCatalogVocabulary
if you want to use alternative widgets. The following example uses Checkboxes:
1from plone.app.vocabularies.catalog import StaticCatalogVocabulary
2from plone.autoform import directives
3from z3c.form.browser.checkbox import CheckBoxFieldWidget
4from zope import schema
5
6uuid_list_field_checkbox = schema.List(
7 title="RelationList with Checkboxes storing uuids",
8 vocabulary=StaticCatalogVocabulary(
9 {
10 "portal_type": "Document",
11 "review_state": "published",
12 }
13 ),
14 required=False,
15)
16directives.widget(
17 "uuid_list_field_checkbox",
18 CheckBoxFieldWidget,
19)
Note
For control panels this is the best way to store relations since you cannot store RelationValue
objects in the registry.
31.8. The stack#
Relations are based on zc.relation. This package stores transitive and intransitive relationships. It allows complex relationships and searches along them. Because of this functionality, the package is a bit complicated.
The package zc.relation
provides its own catalog, a relation catalog.
This is a storage optimized for the queries needed.
zc.relation
is sort of an outlier with regards to Zope documentation. It has extensive documentation, with a good level of doctests for explaining things.
You can use zc.relation
to store the objects and its relations directly into the catalog.
But the additional packages that make up the relation functionality don't use the catalog this way.
We want to work with schemas to get auto generated forms. The logic for this is provided by the package z3c.relationfield. This package contains the RelationValue object and everything needed to define a relation schema, and all the code that is necessary to automatically update the catalog.
A RelationValue Object does not reference all objects directly.
For the target, it uses an id it gets from the IntId
Utility. This id allows direct recovery of the object. The source object stores it directly.
Widgets are provided by plone.app.z3cform
and some converters are provided by plone.app.relationfield
.
The widget that Plone uses can also store objects directly.
Because of this, the following happens when saving a relation via a form:
The HTML shows some nice representation of selectable objects.
When the user submits the form, selected items are submitted by their UUIDs.
The Widget retrieves the original object with the UUID.
Some datamanager gets another unique ID from an IntID Tool.
The same datamanager creates a RelationValue from this id, and stores this relation value on the source object.
Some Event handlers update the catalogs.
You could delete a Relation like this delattr(rel.from_object, rel.from_attribute)
This is a terrible idea by the way, because when you define in your schema that one can store multiple RelationValues, your Relation is stored in a list on this attribute.
Relations depend on a lot of infrastructure to work.
This infrastructure in turn depends a lot on event handlers being thrown properly.
When this is not the case things can break.
Because of this, there is a method isBroken
which you can use to check if the target is available.
There are alternatives to using Relations. You could instead just store the UUID of an object. But using real relations and the catalog allows for very powerful things. The simplest concrete advantage is the possibility to see what links to your object.
The built-in linkintegrity feature of Plone 5 is also implemented using relations.
RelationValues#
RelationValue objects have a fairly complete API.
For both target and source, you can receive the IntId, the object and the path.
On a RelationValue, the terms source
and target
aren't used. Instead, they are from
and to
.
So the API for getting the target is:
to_id
to_path
to_object
In addition, the relation value knows under which attribute it has been stored as from_attribute
. It is usually the name of the field with which the relation is created.
But it can also be the name of a relation that is created by code, e.g. linkintegrity relations (isReferencing
) or the relation between a working copy and the original (iterate-working-copy
).